C development and patching for P01/P59
Re: C development and patching for P01/P59
Ok, so I'm back at this and I've finally been able to compile a simple NOP and a couple basic functions. It was helpful to take a break and do some more learning on programming in general. Now a lot more of this thread makes sense and I can try to tackle a project with this information.
Now I'm trying to decide what I want to do next.....
One question I have is can I create a function (or something else perhaps) that doesn't return?
This may sound a little weird to want, but I would like to be able to create some ASM code that will be patched inline into the PCM OS and continue on without returning.
Now I'm trying to decide what I want to do next.....
One question I have is can I create a function (or something else perhaps) that doesn't return?
This may sound a little weird to want, but I would like to be able to create some ASM code that will be patched inline into the PCM OS and continue on without returning.
LS1 Boost OS V3 Here. For feature suggestions post in here Development Thread. Support future development ->Patreon.
- antus
- Site Admin
- Posts: 8253
- Joined: Sat Feb 28, 2009 8:34 pm
- cars: TX Gemini 2L Twincam
TX Gemini SR20 18psi
Datsun 1200 Ute
Subaru Blitzen '06 EZ30 4th gen, 3.0R Spec B - Contact:
Re: C development and patching for P01/P59
the OS is single threaded, and does not provide memory management. The Pcmhammer flash kernel is a good example of this. Technically you could add a task to one of the scheduler loops but you need to be sure your not going to overload the cpu in a particular worst case scenario when the factory code hits its slowest path at runtime. It would still need to return though as the loop just fires off each task one after the other until it returns.
Have you read the FAQ? For lots of information and links to significant threads see here: http://pcmhacking.net/forums/viewtopic.php?f=7&t=1396
Re: C development and patching for P01/P59
well.. at some point you will want it to return so that the ECU can continue its other operations.. lets make an example here:bubba2533 wrote:Ok, so I'm back at this and I've finally been able to compile a simple NOP and a couple basic functions. It was helpful to take a break and do some more learning on programming in general. Now a lot more of this thread makes sense and I can try to tackle a project with this information.
Now I'm trying to decide what I want to do next.....
One question I have is can I create a function (or something else perhaps) that doesn't return?
This may sound a little weird to want, but I would like to be able to create some ASM code that will be patched inline into the PCM OS and continue on without returning.
GoToStartOfLoop
move.l #$FFFA77, A0
move.w #$1122, D1
move.w (D1),(A0)
cmpi.b #$80,D1
beq.w GoToSubRoutine
bra.w GoToStartOfLoop
GoToSubRoutine
... continue ASM here..
In terms of patching and making "room". I have found that you can either:
1) remove a line or two and optimise to create space. For example, the above could have just done this:
move.l #$FFFA77, A0
move.w #$1122,(A0)
cmp.b (A0),D1
We have condensed a single line, that was not being used anywhere else. This then allows us to then add ASM in to jump else where:
move.l #$FFFA77, A0
bsr.w GoToMyCustomRoutine
move.w #$1122,(A0)
cmp.b (A0),D1
..... (lots of asm here)
GoToMyCustomRoutine
.. do something cool here
rts (return)
2) Remove a line or two from a function, add a jump to empty space of code, then add the line or two removed for the custom jump into the custom function.
Both of the above examples require returning back to the original PCM coding since.. well.. we need it to do its job still and run the entire car. These are just methods of patching the code without changing the size of the bin. This is important for places in memory which use a JSR command, as they take a raw address to jump to, thus if the bin size changes, this will affect the address it jumps to.
Your Local Aussie Reverse Engineer
Contact for Software/Hardware development and Reverse Engineering
Site:https://www.envyouscustoms.com
Mob:+61406 140 726
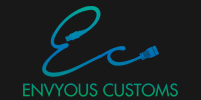
Contact for Software/Hardware development and Reverse Engineering
Site:https://www.envyouscustoms.com
Mob:+61406 140 726
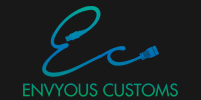
Re: C development and patching for P01/P59
All of that seems way over my head and not something that I want to mess with. I probably should have provided more info so you could understand what I was wanting to do.antus wrote:the OS is single threaded, and does not provide memory management. The Pcmhammer flash kernel is a good example of this. Technically you could add a task to one of the scheduler loops but you need to be sure your not going to overload the cpu in a particular worst case scenario when the factory code hits its slowest path at runtime. It would still need to return though as the loop just fires off each task one after the other until it returns.
Here is a very simple example of an instruction that I would want to disable/change:
42 38 B5 56 // clr.b (0xFFB556)
So I need something that takes up the space of 4 bytes. a "nop" is 2 bytes so I wrote this simple function:
Code: Select all
void DoNothing()
{
asm("nop");
asm("nop");
}
Bytes // asm operation
4E 71 // nop
4E 71 // nop
4E 75 // rts
Well, the DoNothing() won't work because it produces 6 bytes including the "rts" that I didn't want.
Obviously for this simple instruction I could just change it manually, but then where is the fun in that. I was hoping I could provide something "inline" to remove the "rts" operation. But doing that seems to just not output anything because I'm not using the function anywhere.
It's probably not worth figuring out, but I thought I would ask.
LS1 Boost OS V3 Here. For feature suggestions post in here Development Thread. Support future development ->Patreon.
- antus
- Site Admin
- Posts: 8253
- Joined: Sat Feb 28, 2009 8:34 pm
- cars: TX Gemini 2L Twincam
TX Gemini SR20 18psi
Datsun 1200 Ute
Subaru Blitzen '06 EZ30 4th gen, 3.0R Spec B - Contact:
Re: C development and patching for P01/P59
the rts is required to control the program flow, you cant have the function return any other way. if you are replacimg enough code eg something like you replace a block of egr code you can put your new code over existing code and not have the jmp to your function or the rts but you then need to make sure your patch is exactly the right size and pad it with nops to fit the bit you are removing.
Have you read the FAQ? For lots of information and links to significant threads see here: http://pcmhacking.net/forums/viewtopic.php?f=7&t=1396
Re: C development and patching for P01/P59
That's only if I jump to the function. I want to place the function inline.
I'm aware I need to make it the same size. I've made dozens of patches manually, but I am hoping to try to automate some part of it. That way I can iterate quickly and try different code.
I'm aware I need to make it the same size. I've made dozens of patches manually, but I am hoping to try to automate some part of it. That way I can iterate quickly and try different code.
LS1 Boost OS V3 Here. For feature suggestions post in here Development Thread. Support future development ->Patreon.
Re: C development and patching for P01/P59
You are going to need to be creative with batch/scripts/etc...
Intelligence is in the details!
It is easier not to learn bad habits, then it is to break them!
If I was here to win a popularity contest, their would be no point, so I wouldn't be here!
It is easier not to learn bad habits, then it is to break them!
If I was here to win a popularity contest, their would be no point, so I wouldn't be here!
Re: C development and patching for P01/P59
Yeah I was thinking that might be the only option for the inline modifications that I have to do.Gampy wrote:You are going to need to be creative with batch/scripts/etc...
I hit another issue that I can't seem to find a solution for.
I know that C will likely be less efficient at handling things compared to the ASM version that I already have. So I was just going to copy my current ASM into a C wrapper function to try and get everything in a better format.
I'm stuck on an issue that will mostly kill that idea. I was hoping to define a lot of the ram addresses for common variables in the header (like Manifold_Pressure = 0xFFAEEC) and be able to use it in my assembly which would make it more readable.
I am able to get the following code to compile:
Code: Select all
void update_map()
{
asm (
"lsr.w #1,%d3;"
"move.w %d3,(0xFFFFAEEC).w;"
"move.w (0xFFFFB292),%d3;"
"cmp.w #400,%d3;"
);
}
If anyone has ideas (or more reference material) I'm willing to try them :thumbup:
LS1 Boost OS V3 Here. For feature suggestions post in here Development Thread. Support future development ->Patreon.
Re: C development and patching for P01/P59
Scratch that...I'm going to try and write it in native ASM and try to compile it...fingers crossed
not sure why I didn't think of that
not sure why I didn't think of that
LS1 Boost OS V3 Here. For feature suggestions post in here Development Thread. Support future development ->Patreon.
- antus
- Site Admin
- Posts: 8253
- Joined: Sat Feb 28, 2009 8:34 pm
- cars: TX Gemini 2L Twincam
TX Gemini SR20 18psi
Datsun 1200 Ute
Subaru Blitzen '06 EZ30 4th gen, 3.0R Spec B - Contact:
Re: C development and patching for P01/P59
I would use asm, I dont think C is worth it unless your skills in C for an embedded target are very strong. Even the pcmhammer kernel is way bigger than it should be because we had to turn off optimisation because the we didnt have the embedded syntax right for the whole app and the compiler was optimising out code that needed to be there because it was talking to raw hardware. you can use the volatile keyword to tell the compiler that it cant trust that data in that location is not changing on its own, but its more than that to be able to turn on optimisation in the pcmhammer kernel, and we never got it right. the outcome is without optimisation the asm it creates is wasteful and huge, it should be half the size.
Have you read the FAQ? For lots of information and links to significant threads see here: http://pcmhacking.net/forums/viewtopic.php?f=7&t=1396